Astro 项目优化技巧
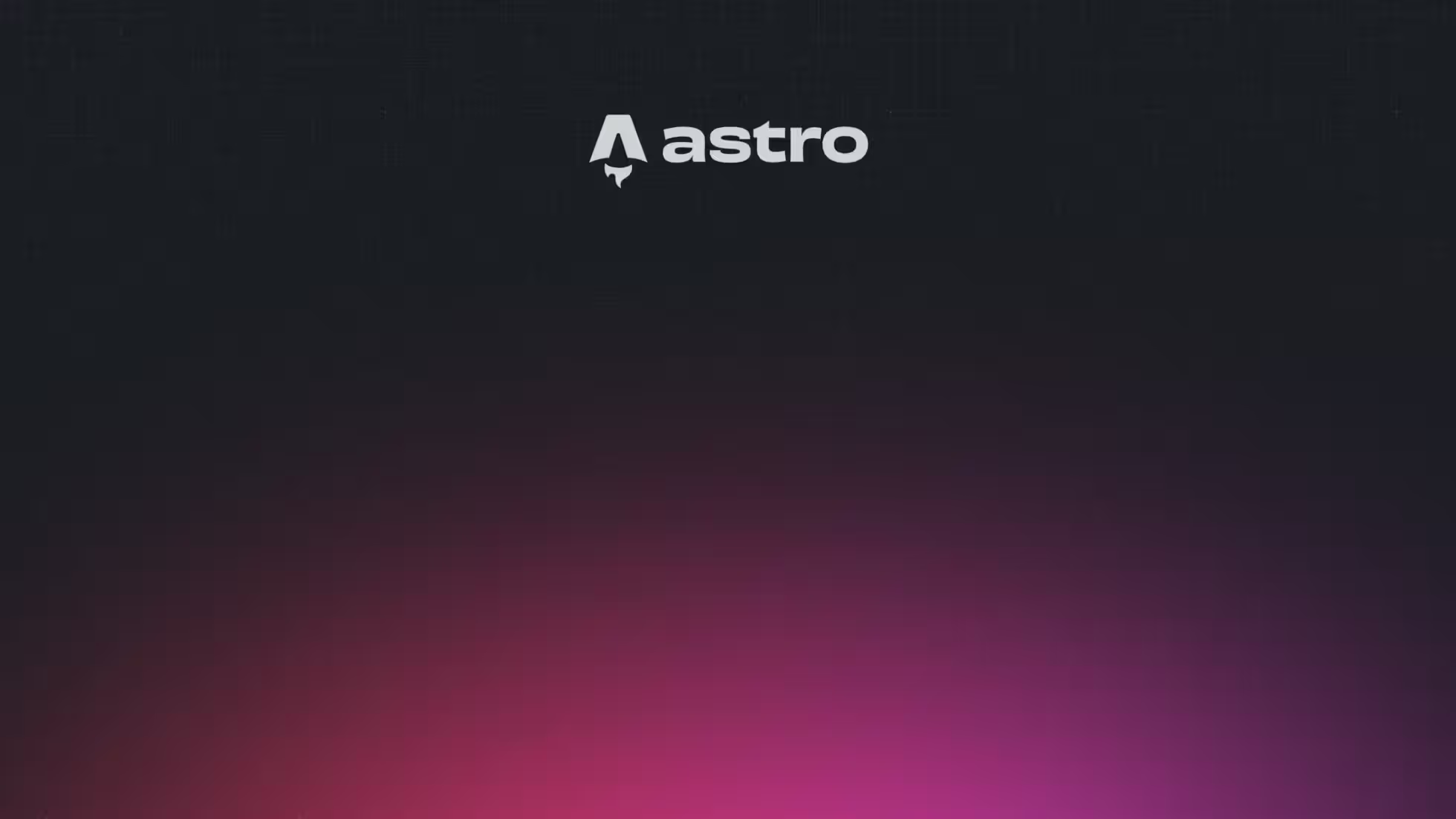
清理和重建项目
清理和重建项目有时可以解决一些缓存或编译问题。
- 停止开发服务器:如果你的开发服务器正在运行,请先停止它。 Mac 使用 control+c 停止。
- 删除缓存目录:根据项目配置和使用的工具,可能会有一个或多个缓存目录。通常是
node_modules/.cache/
和.astro/
目录。 - 重新安装依赖项:运行 npm install 或
yarn
来确保所有依赖项都正确安装。 - 重新启动开发服务器:运行
npm run dev
或yarn dev
来重新启动你的开发服务器。 - 重新构建项目:如果想构建项目的生产版本,可以运行
npm run build
或yarn build
。
以下是在命令行中执行这些步骤的示例命令:
# Stop the development server if it's running
# Delete cache directories
rm -rf node_modules/.cache/
rm -rf .astro/
# Reinstall dependencies
npm install
# Restart the development server
npm run dev
# (Optional) Build the project for production
# npm run build
代码格式检查
1. 安装 Prettier
如果项目还没有安装 Prettier,你可以通过以下命令来安装: 使用 npm:
npm install --save-dev prettier
使用 pnpm:
pnpm add --save-dev prettier
2. 检查代码格式
你可以使用以下命令来检查代码的格式:
npx prettier --check "./path/to/your/files/**/*.js"
替换 "./path/to/your/files/**/*.js"
为想要检查的文件或目录的路径。
3. 自动修复代码格式
可以使用以下命令来自动修复代码格式:
npx prettier --write "./path/to/your/files/**/*.js"
同样,替换 "./path/to/your/files/**/*.js"
为想要修复的文件或目录的路径。
4. (可选)配置 Prettier
如果想自定义 Prettier 的行为,你可以在项目根目录下创建一个 .prettierrc
文件,并在其中添加配置。例如:
{
"singleQuote": true,
"semi": false
}
这将配置 Prettier 使用单引号并省略分号。
5. (可选)集成到代码编辑器
许多代码编辑器(如 VS Code)有 Prettier 插件,可以在你保存文件时自动格式化代码。你可以查看你所使用的编辑器的插件或扩展商店,搜索并安装 Prettier 插件。
固定链接 (Permalink)
Astro的内容集合(Content Collections)是Astro项目中管理和创建内容的最佳方式。内容集合用于组织文档,并验证前端内容。
内容集合是在保留的 src/content
项目目录中的顶级目录,例如 src/content/newsletter
和 src/content/authors
。只有内容集合才能位于 src/content
目录中,该目录不能用于其他任何目的。
在Astro中,每个内容条目都会从其文件ID生成一个URL友好的slug属性。这个slug用于直接从集合中查询条目,也用于从内容创建新的页面和URL。
类似于其他Web框架的“永久链接(Permalink)”,可以通过在文件的frontmatter中添加自己的slug属性来覆盖条目的生成的slug。“slug”是一个特殊的、受保留的属性名,在自定义集合模式中不允许使用,并且不会出现在条目的数据属性中。
例如:
---
title: My Blog Post
slug: my-custom-slug/supports/slashes
---
Your blog post content here.
在这个例子中,通过在frontmatter中定义slug属性,可以为特定的博客文章设置自定义的URL路径。这意味着可以完全控制URL的结构,并按照自己的需求组织内容。
比如可以使用这种方法来实现将URL从 http://localhost:3000/blog/markdown-style-guide/
改为 http://localhost:3000/markdown-style-guide/
。
-
更新每个博客文章的frontmatter:在每个博客文章文件的frontmatter中,添加一个slug属性,该属性包含不带
/blog/
前缀的自定义路径。--- title: Markdown Style Guide slug: markdown-style-guide --- Your blog post content here.
-
配置动态路由文件:
[...slug].astro
是一个动态路由文件,其中slug是变量,可以匹配任何值,用于处理文章的slug。把这个文件放置在src/pages,而不是对应src/pages/blog 子文件中。 -
获取文章集合:代码中使用
await getCollection('blog')
获取名为”blog”的文章集合。 -
静态路径生成:
getStaticPaths
函数通过映射文章的slug生成静态路径。 -
文章渲染:文件的其余部分用于渲染特定的文章内容,包括相关文章、目录等。
---
import { type CollectionEntry, getCollection } from "astro:content";
import BlogPost from "@/layouts/BlogPost";
const posts = await getCollection("blog");
export async function getStaticPaths() {
const posts = await getPosts();
return posts.map((post) => ({
params: { slug: post.slug },
props: post,
}));
}
type Props = CollectionEntry<"blog">;
const post = Astro.props;
const { Content, headings, remarkPluginFrontmatter } = await post.render();
---
<BlogPost
data={post.data}
headings={headings}
readTime={remarkPluginFrontmatter.minutesRead}
>
<div
class="mt-8 flex flex-col justify-center gap-16 px-1 sm:px-4 md:flex-row md:pr-12"
>
<div class="mx-auto w-full max-w-full md:w-4/5">
<div class="prose-wrapper mx-auto max-w-screen-md">
<div class="prose mb-12 md:prose-lg dark:prose-invert">
<Content components={{ pre: Code }} />
</div>
</div>
</div>
</div>
</BlogPost>
启用阅读时长
在 Astro 项目中启用阅读时长的功能可以增强用户体验,让读者了解每篇文章的大致阅读时间。以下是在 Astro 静态中文汉语网站项目中启用阅读时长的方法
1. 安装依赖
首先,你需要安装 reading-time
包,用于计算阅读时长。
使用 pnpm,运行以下命令:
pnpm add reading-time
2. 创建阅读时长插件
在项目的源码目录中,创建一个文件,例如 remarkReadingTime.js
,并将以下代码粘贴到文件中:
import getReadingTime from 'reading-time'
import { toString } from 'mstringdast-util-to-'
export function remarkReadingTime() {
return function (tree: unknown, { data }: any) {
const textOnPage = toString(tree)
const readingTime = getReadingTime(textOnPage, { wordsPerMinute: 333 })
const minutes = Math.ceil(readingTime.minutes)
const timeText = minutes + ' 分钟'
data.astro.frontmatter.minutesRead = timeText
}
}
这段代码定义了一个用于计算阅读时长的 remark 插件。
3. 在 Astro 配置中使用插件
接下来,你需要在 Astro 的配置文件中使用此插件。打开 astro.config.mjs
文件,并修改 markdown 的配置,以便包括你刚刚创建的插件。
import { remarkReadingTime } from "./src/utils/remarkReadingTime";
export default defineConfig({
markdown: {
remarkPlugins: [remarkReadingTime],
},
// 其他配置...
});
确保正确导入了你的插件,并将其添加到 remarkPlugins
数组中。
4. 在页面中显示阅读时长
在 Astro 组件或页面中,你可以通过 frontmatter.minutesRead
访问计算出的阅读时长,并将其渲染到页面上。
---
import { Markdown } from "astro/components";
const content = `# 你的 Markdown 内容`;
const readingTime = content.frontmatter.minutesRead;
---
<article>
<Markdown content={content} />
<footer>阅读时长:{readingTime}</footer>
</article>
使用 Waline 作为评论系统
1. 创建 Waline 组件
首先,创建一个名为 WalineComment.astro
的专门组件,用于封装 Waline 的评论框和相关代码。
在 WalineComment.astro
文件中:
<div id="waline"></div>
<script type="module">
import { init } from "https://unpkg.com/@waline/client@v2/dist/waline.mjs";
document.querySelector("#waline").addEventListener("load", () => {
init({
el: "#waline",
serverURL: "https://comment.domain.com", // 你的 Waline 服务器地址
});
});
</script>
<style>
:root {
--waline-theme-color: #737373; /* 新的主题颜色 */
--waline-active-color: #404040; /* 新的活动颜色 */
}
</style>
2. 在页面中嵌入 Waline 组件
假设你想在博客文章页面中添加 Waline 评论框。在你的文章页面组件(例如 src/pages/[...slug].astro
)中,你可以如下引入并使用 WalineComment
组件:
---
import WalineComment from "@/components/WalineComment";
---
<div class="w-full max-w-full">
<div class="markdown prose mb-12 dark:prose-invert">
<Content components={{ pre: Code }} />
</div>
<!-- 插入 Waline 评论组件 -->
<WalineComment />
</div>
3. 样式链接
确保你在全局布局组件的 <head>
部分添加了 Waline 的样式链接:
<head>
<link
rel="stylesheet"
href="https://unpkg.com/@waline/client@v2/dist/waline.css"
/>
</head>
- 统计文章阅读量
首先在Waline 组件中启用pageview: true
,然后在需要展示页面阅读量的地方插入
共 <span class='waline-pageview-count'></span> 次浏览
。
百度统计
Partytown 是一个用于改善网站性能的库,通过将第三方脚本的执行转移到 Web Worker 中,降低主线程的工作负担。
-
安装 Partytown: 如果还没有安装 Partytown,首先通过 npm 或 yarn 安装它。
npm install @astrojs/partytown # 或 yarn add @astrojs/partytown
-
配置 Partytown: 在Astro 配置文件 (
astro.config.mjs
) 中,添加 Partytown 作为插件,并配置需要转发的全局变量。import partytown from "@astrojs/partytown"; export default defineConfig({ // 其他配置 integrations: [ // 其他插件 partytown({ config: { forward: ["_hmt"], // 添加需要转发的全局变量 }, }), ], });
-
修改百度统计代码: 在 Astro 文件中,将百度统计的代码修改为使用 Partytown。只需将原始的
<script>
标签的type
属性更改为text/partytown
即可。<script type="text/partytown"> var _hmt = _hmt || []; (function() { var hm = document.createElement("script"); hm.src = "https://hm.baidu.com/hm.js?b436228ed0052709b87aff438828425a"; var s = document.getElementsByTagName("script")[0]; s.parentNode.insertBefore(hm, s); })(); </script>